Handling exceptions when triggering the SAP BTP API
In this SAP note, I would like to describe how a developer can catch different types of exceptions for the API to prevent it from failing or making unnecessary calls that will fail with an error. SAP BTP API Management has various standard tools that will allow me to achieve the desired results.
Scenario
For a newly created API, it's essential to check if the mandatory header parameters have been provided for the API call. The required parameters are:
- startDate
- endDate
Additionally, I need to ensure that the date range provided does not exceed seven days. If one of the header parameters is missing or the date range exceeds one week, the API should trigger an exception with the response code 999 - Invalid Header Params. Otherwise, the API call should proceed as expected.
API
For simplicity, I will create the API based on the well-known URL.
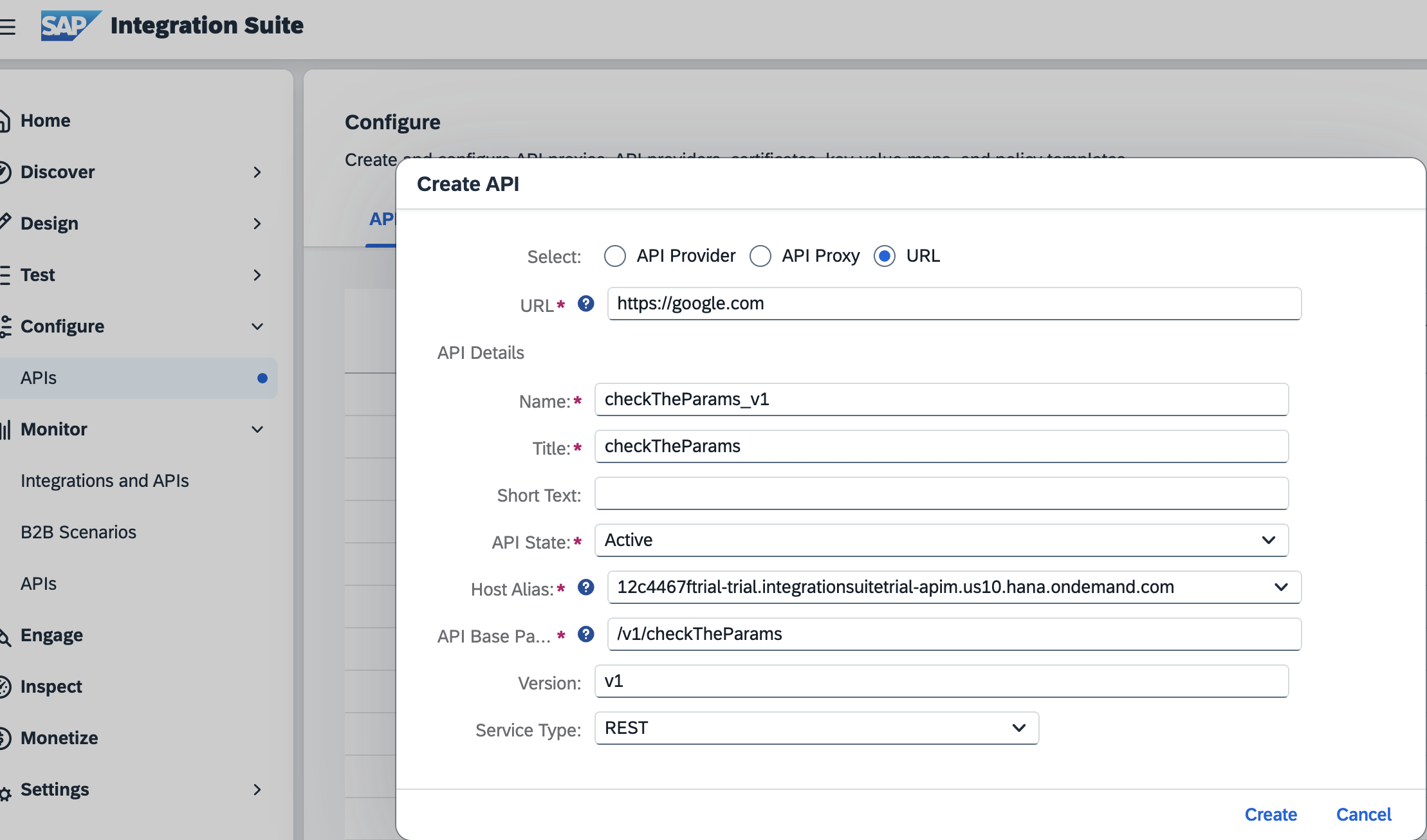
Check the required params for the API call
To check if the mandatory header parameters have been provided, I will create a new Policy with JavaScript code inside it.
See JavaScript
See Java Script Object Model
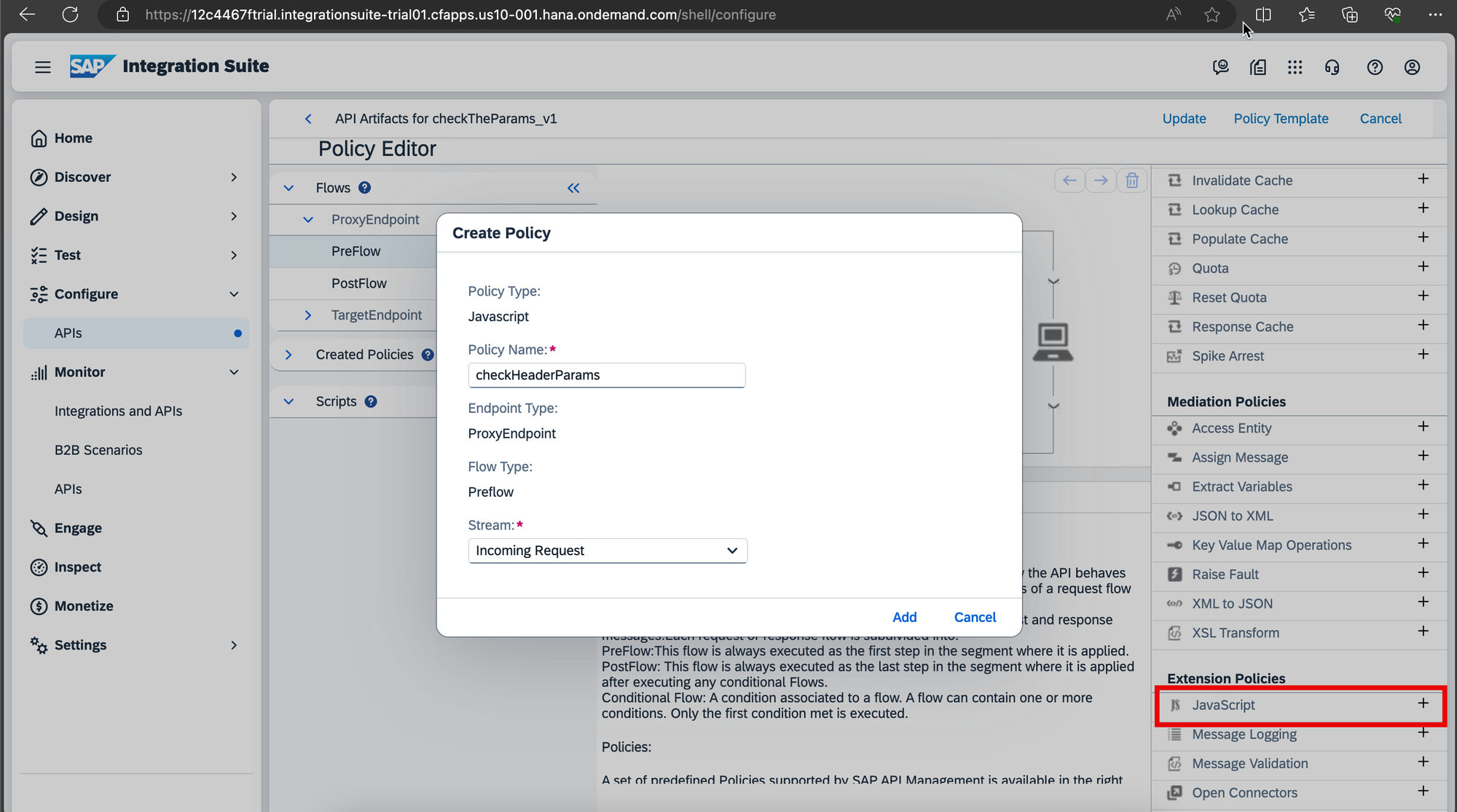
Below is an example of the code:
var startDate = context.getVariable("request.header.startDate");
var endDate = context.getVariable("request.header.endDate");
if (startDate && endDate) {
}
else{
context.setVariable("validation.isErrorFound", true);
}
Add the Raise Fault policy
Now, I can apply another policy that will be triggered if not all the mandatory parameters are present in the header.
See Raise Fault
See Condition Strings
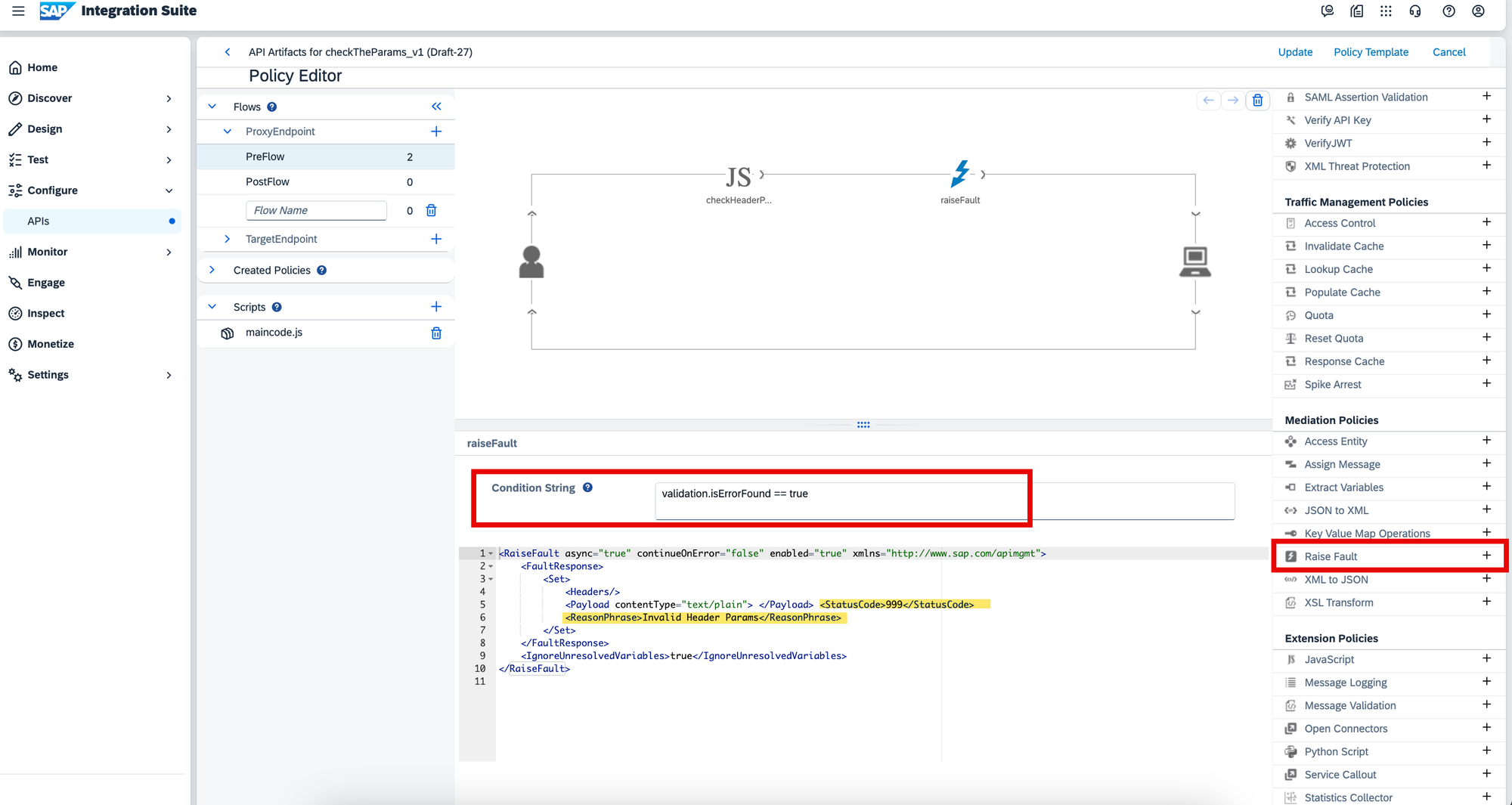
Please note that the Raise Fault policy should only be triggered under certain conditions.
validation.isErrorFound == true
Save and deploy the changes.
Interim testing
Let's test the API's behavior after implementing the new policies.
Enhancing the JavaScript policy
Now we are ready to implement one more condition into the JavaScript policy, which will be used to determine the duration of the period given in the header.
var startDate = context.getVariable("request.header.startDate");
var endDate = context.getVariable("request.header.endDate");
function dateDifferenceInDays(start, end) {
var startDate = new Date(start);
var endDate = new Date(end);
var timeDiff = endDate.getTime() - startDate.getTime();
var diffDays = timeDiff / (1000 * 3600 * 24);
return diffDays;
}
if (startDate && endDate) {
var diff = dateDifferenceInDays(startDate, endDate);
if (diff > 7) {
context.setVariable("validation.isErrorFound", true);
}
}
else{
context.setVariable("validation.isErrorFound", true);
}
I understand that the above code could be improved to encompass more validations, but I am using it to provide an idea of what could be done. Please feel free to enhance it as required for your business needs.
Final testing
Perform the final testing of the implemented validations for the API.