Creating a Web Application Using the SAPUI5 Framework (Part 2)
Creating a Web Application Using the SAPUI5 Framework (Part 2)
After creating the skeleton of my application, I’ll try to make it a bit more dynamic by involving some visual elements and a touch of mechanics. To maintain continuity with the previous step:
See the SAP note: Creating a Web Application Using the SAPUI5 Framework (Part 1)
The visual part with a touch of mechanics, as mentioned above, will be implemented through the active use of the View and Controller. There is a detailed and well-written reference guide on the MVC concept used in SAPUI5:
See: Model View Controller (MVC)
See: Model
See: View
See: Controller
Controller
Just for fun, I’ll define the onInit()
function, which will show a non-intrusive message when the application is initially loaded.
onInit(): Called when a view is instantiated and its controls (if available) have already been created; used to modify the view before it is displayed, bind event handlers, and perform other one-time initialization. \
Path to the controller: /webapp/controller/Default.controller.js
The changes mentioned:
onInit: function () {
var message = 'onInit function successfully loaded';
MessageToast.show(message);
}
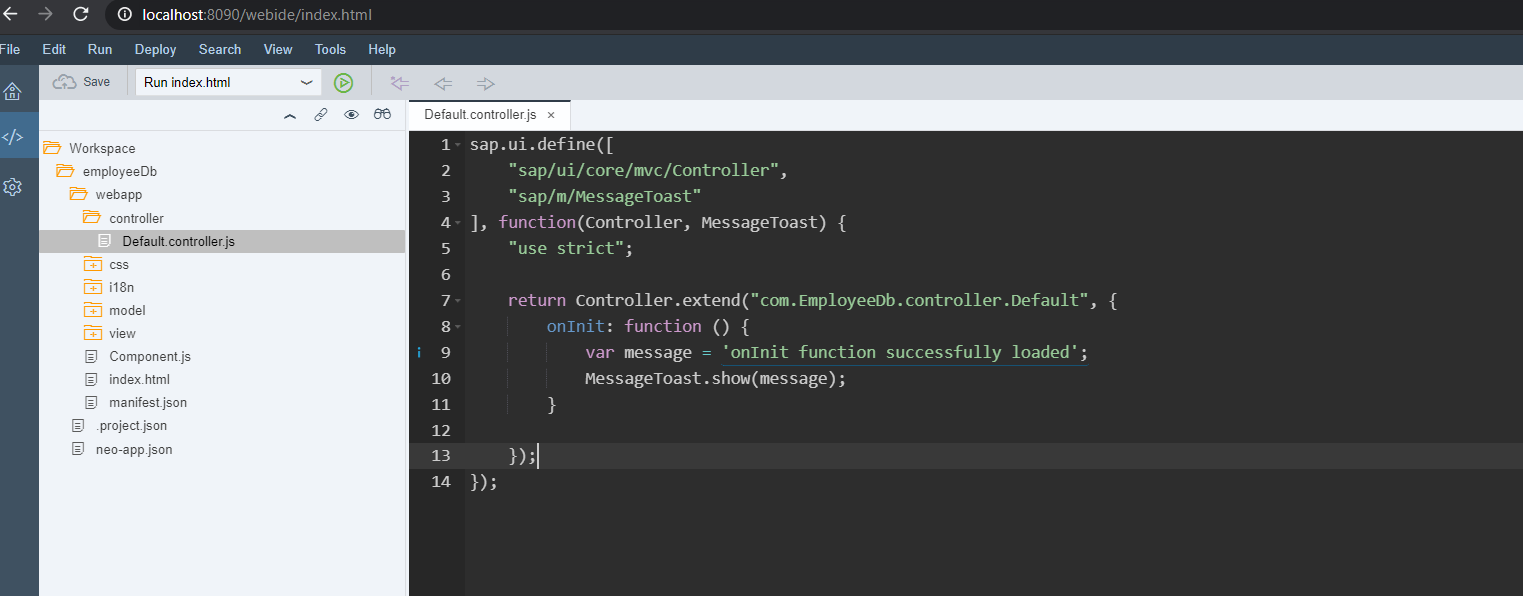
Trying to run it.
View
Previously, I defined one View for my application, where I will later introduce the visual changes I need. You can explore the library of all possible UI elements on the main reference portal for the framework:
See: Samples
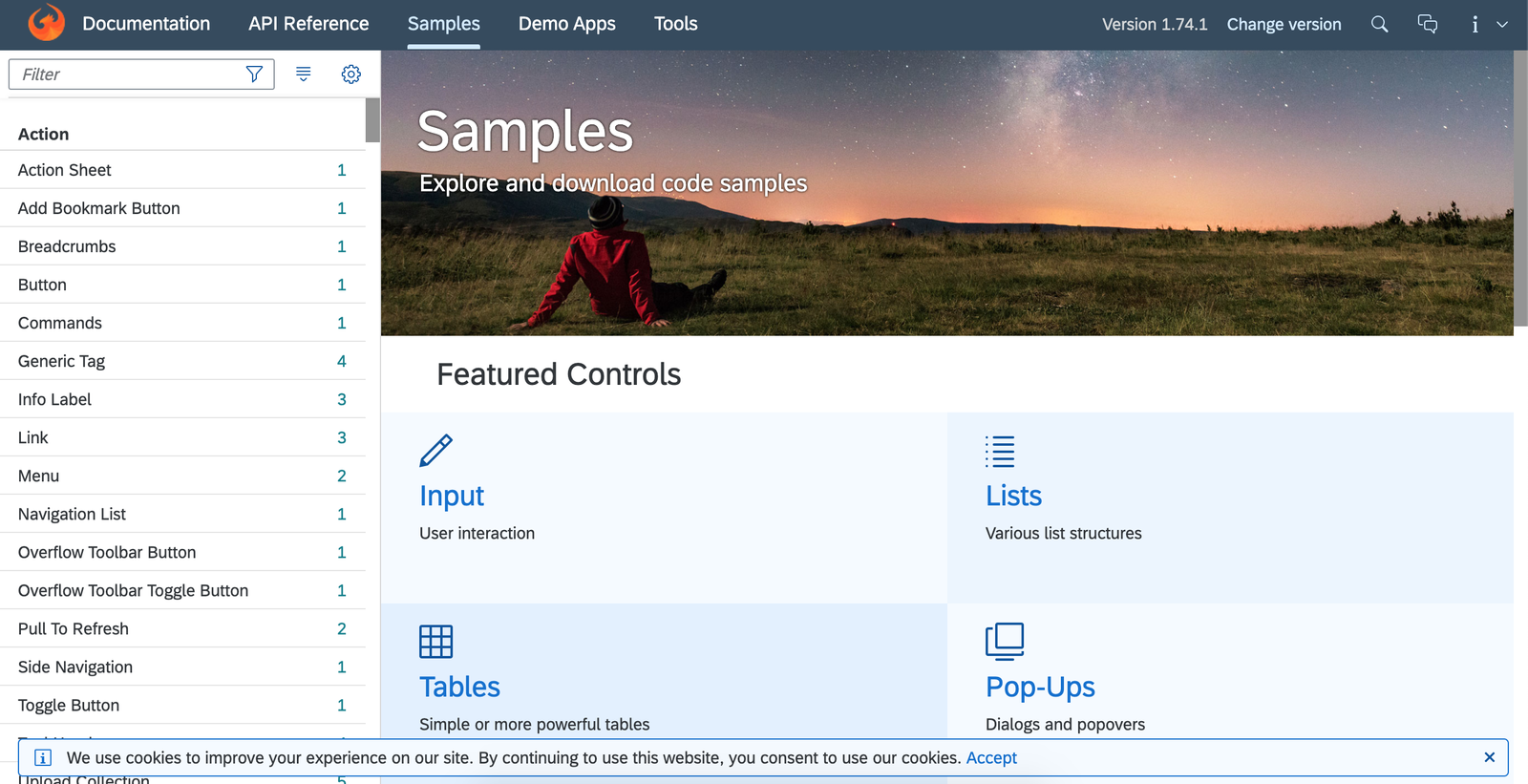
Using this library, you can:
- Quickly find and review all available elements you might use in your application
- Quickly get an idea of how the elements will look
- View the source code for the view and controller (this point seems especially valuable to me)
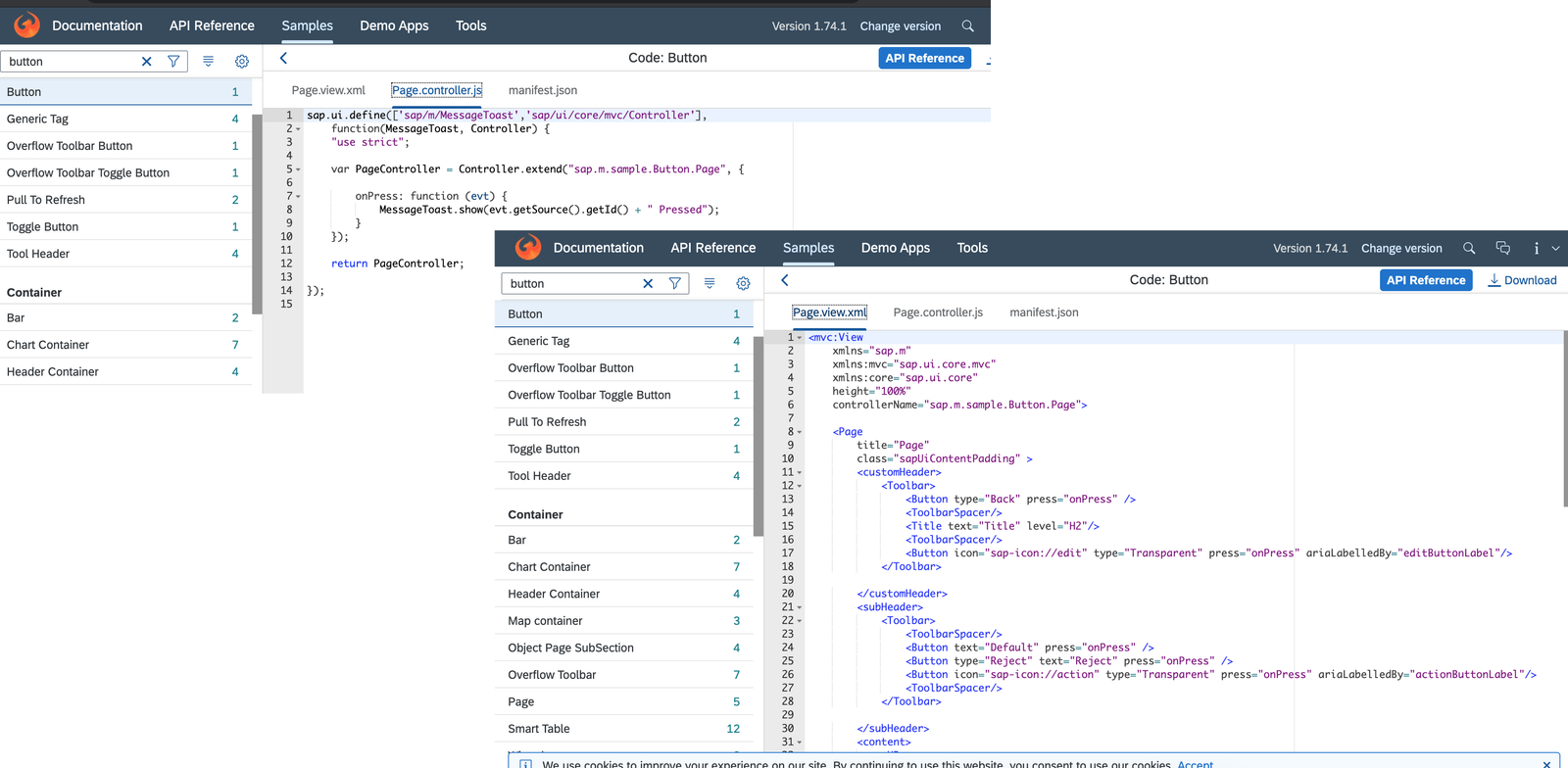
To demonstrate this note, I’ll use the StandardListItem
element:
<mvc:View controllerName="com.EmployeeDb.controller.Default" xmlns:html="http://www.w3.org/1999/xhtml" xmlns:mvc="sap.ui.core.mvc"
displayBlock="true" xmlns="sap.m">
<App>
<pages>
<Page title="{i18n>title}">
<content>
<List id="idList">
<StandardListItem
title="test"
description="test"/>
<StandardListItem
title="test2"
description="test2"/>
<StandardListItem
title="test3"
description="test3"/>
</List>
</content>
</Page>
</pages>
</App>
</mvc:View>
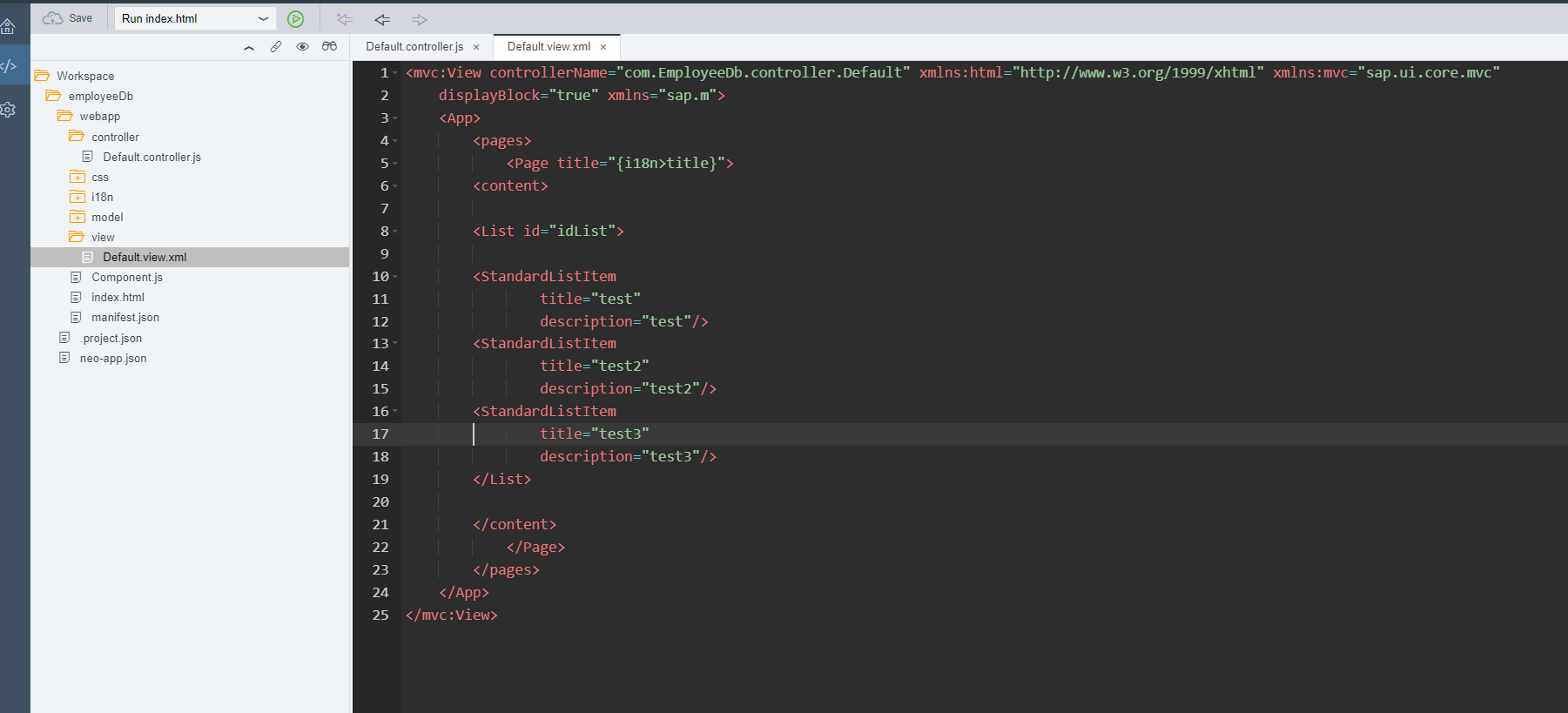
Running the application again.
Still rather useless for now, but there’s already some kind of trend taking shape 🤦. I’ll add a couple more buttons:
<mvc:View controllerName="com.EmployeeDb.controller.Default" xmlns:html="http://www.w3.org/1999/xhtml" xmlns:mvc="sap.ui.core.mvc"
displayBlock="true" xmlns="sap.m">
<App>
<pages>
<Page title="{i18n>title}">
<content>
<List id="idList">
<StandardListItem
title="test"
description="test" />
<StandardListItem
title="test2"
description="test2"/>
<StandardListItem
title="test3"
description="test3"/>
</List>
<Button id="buttonOne" text="First button" press="onPressOne"/>
<Button id="buttonTwo" text="Second button" press="onPressTwo"/>
</content>
</Page>
</pages>
</App>
</mvc:View>
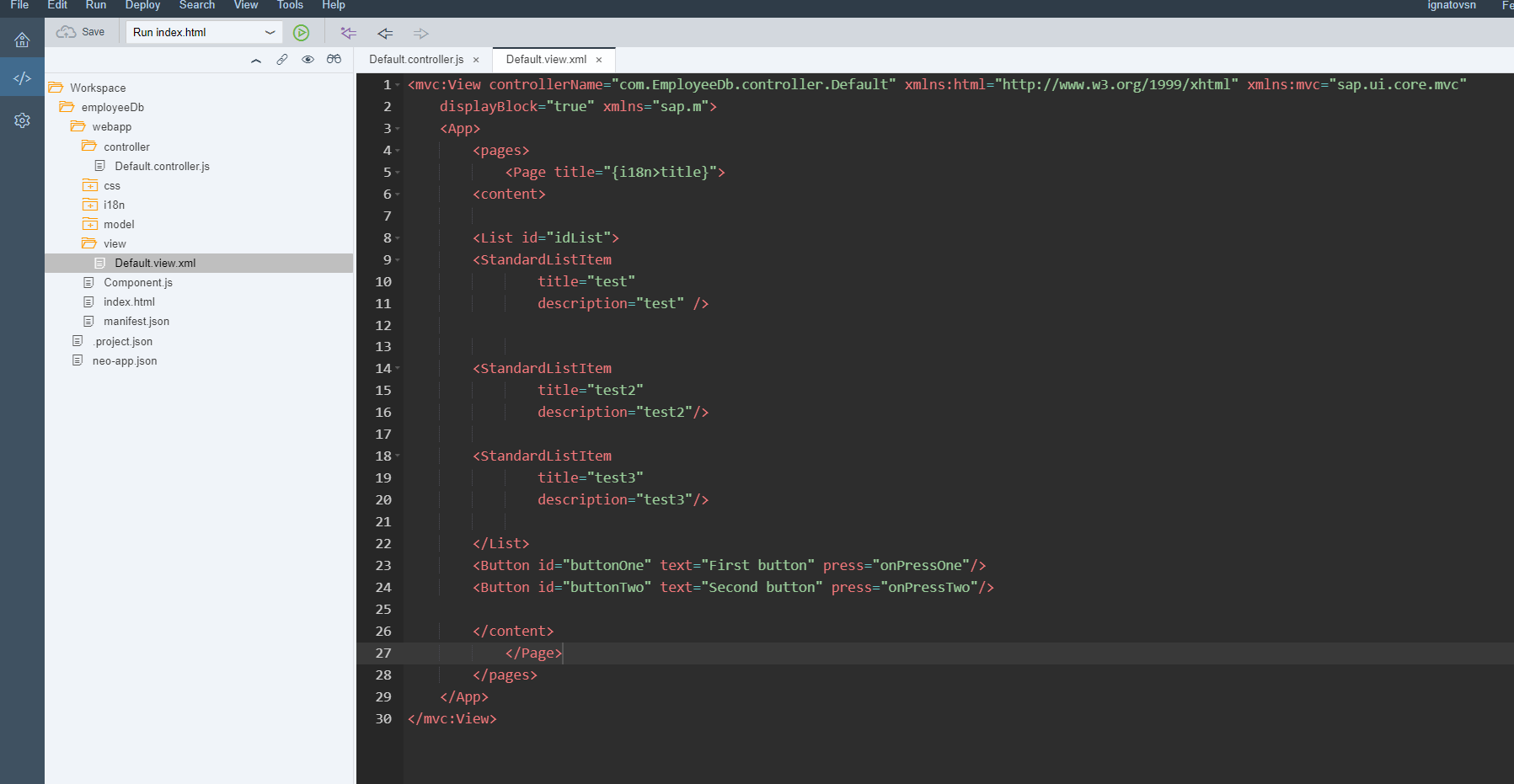
Don’t forget to handle the button press events:
onPressOne: function (oEvent) {
MessageToast.show(oEvent.getSource().getId() + " Pressed");
},
onPressTwo: function (oEvent) {
MessageToast.show(oEvent.getSource().getId() + " Pressed");
}
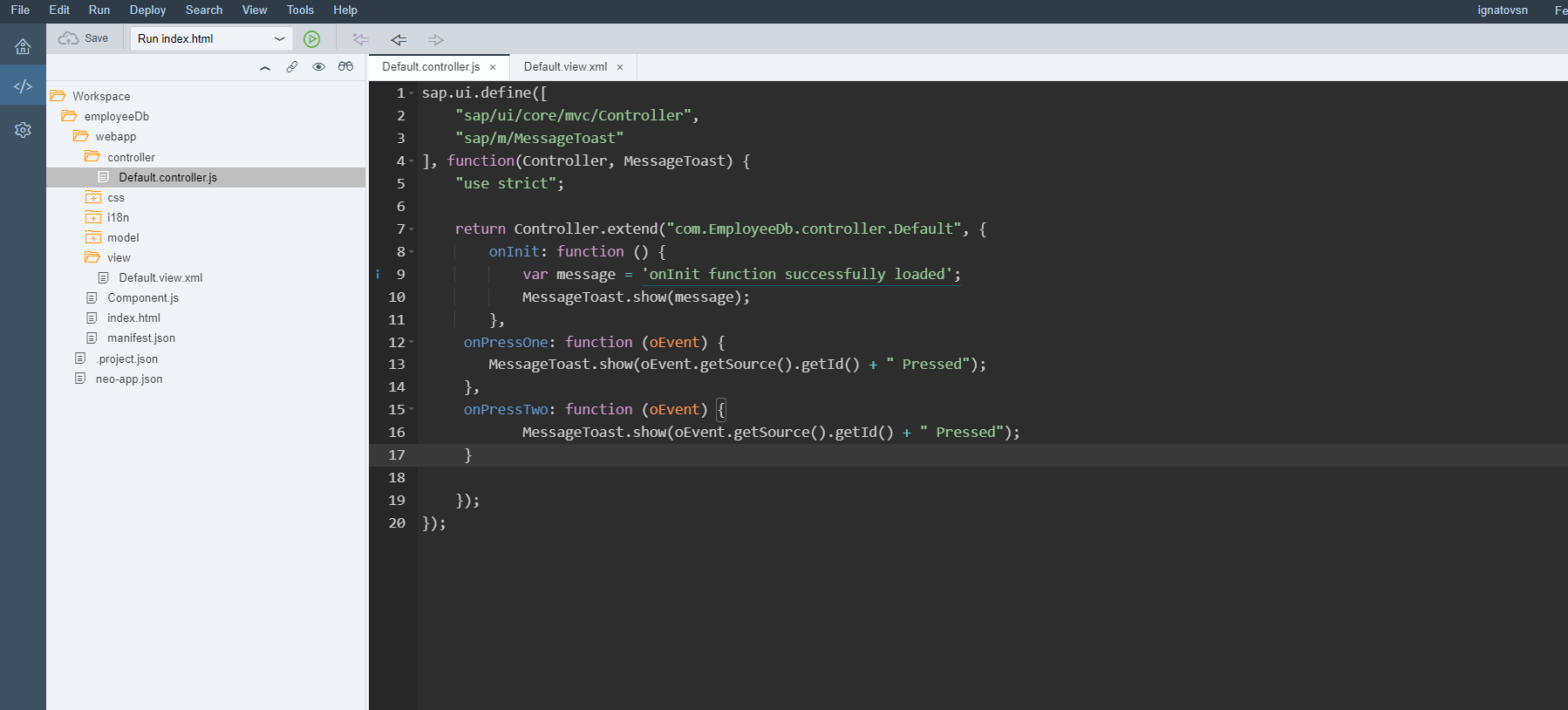
Testing
Thank you for your attention.